Back to blog
Wednesday, April 2, 2025
Creating Custom Code Snippets for Multiple Programming Languages
Posted by
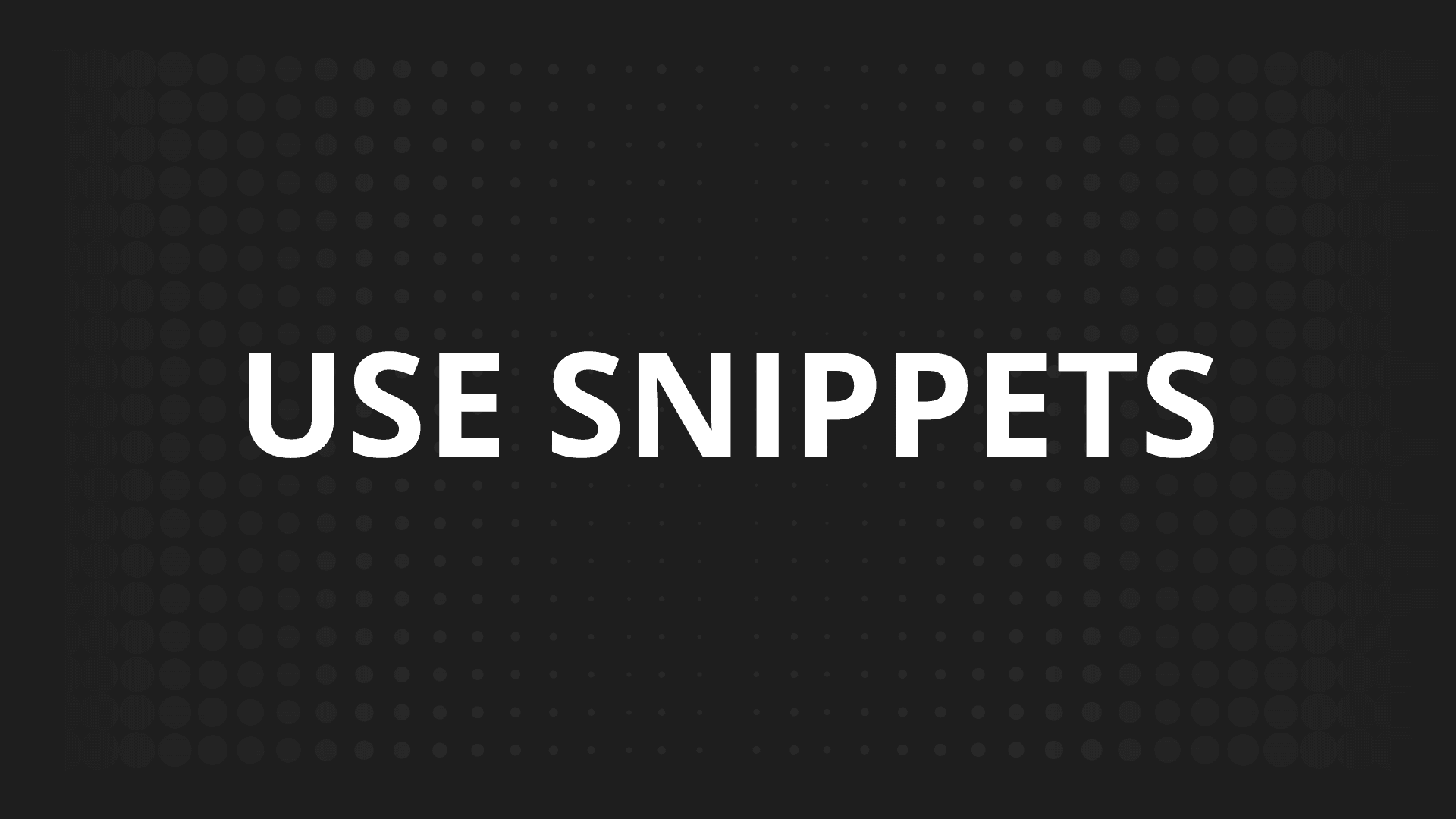
Introduction: Enhancing Productivity with Code Snippets
Code snippets are essential tools for developers, enabling quick reuse of commonly used logic across different projects. In this article, we’ll explore how to create and manage custom code snippets for TypeScript, JavaScript, Java, and Python. These snippets will help streamline development workflows and ensure consistency across projects.
Organizing Code Snippets
A well-structured snippet system should include:
- Language-Specific Snippets: Snippets tailored for each programming language.
- Reusability: Modular and reusable components.
- Consistency: Standardized formatting and naming conventions.
Example Snippets
TypeScript: Utility Function for Deep Cloning Objects
function deepClone<T>(obj: T): T {
return JSON.parse(JSON.stringify(obj));
}
JavaScript: Debounce Function for Optimizing Event Handling
function debounce(func, delay) {
let timer;
return function (...args) {
clearTimeout(timer);
timer = setTimeout(() => func.apply(this, args), delay);
};
}
Java: Singleton Pattern Implementation
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
Python: Context Manager for Timing Code Execution
import time
from contextlib import contextmanager
@contextmanager
def timer():
start = time.time()
yield
end = time.time()
print(f"Execution Time: {end - start:.2f} seconds")
Best Practices for Managing Snippets
- Use Snippet Management Tools: Leverage tools like VSCode snippets, GitHub Gists, or custom repositories.
- Document Each Snippet: Include a short description explaining its purpose and usage.
- Keep Snippets Up-to-Date: Regularly review and update snippets to align with best practices.
Conclusion
Custom code snippets significantly boost efficiency by reducing repetitive coding tasks. By maintaining a well-organized snippet collection, developers can save time and improve code consistency across projects.
🚀 Happy coding!